Attaching internet based webhooks to localhost
This tutorial will show you how you can use webhooks directly from your C# or VB.NET web application, without the need to publish your app to a live server or cloud service. Watch the video or read the tutorial below.
Using webhooks via your local machine
Webhooks play an important role in many web applications. By handling user-defined HTTP callbacks, webhooks can let your application know when a specific event occurs on a 3rd party service. More and more services are offering the ability to use webhooks by allowing you to set a URL for HTTP requests.
Webhooks are available with many SaaS providers such as Twilio, Zapier, Dropbox, SendGrid, PayPal and many more.
However before using these in your web application, you usually need to either publish to a live server or to Azure, so that your application can be called from the internet.
But what if you want to work with webhooks directly, from your local machine?
Perhaps you want to debug your application without having to publish it.To achieve just that, we will use Conveyor to provide incoming access to our web project through a tunnel, enabling your webhooks to be called on localhost. Conveyor is available through the Tools->Extensions menu, by searching for conveyor.
Setup an MVC Web Application
To get started, we’re going to setup a simple webhooks MVC demo app in Visual Studio using C#. We will use the Twilio service to notify our application when a text message (SMS) has been received and respond with a specific message. This tutorial can also be used for Twilio's other API's including Chat, Voice and Video.
This example project is designed to show you how Conveyor can be used to simplify the use of webhooks on your local machine. If you need more details about how to setup webhooks please look online at numerous tutorials and projects.
Create a new project, we’ll be creating an empty ASP.NET MVC C# project. We won’t be hosting in the Cloud so don’t select Azure.
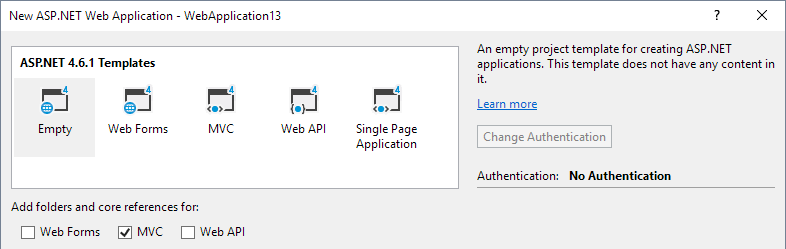
Add NuGet Packages
In order to use the Twilio helper libraries we will need to install the Twilio NuGet package via the Package Manager console. Enter the following line and hit return;
Install-Package Twilio.AspNet.Mvc -DependencyVersion HighestMinor
Create a new Controller
To listen for the Twilio webhook we’ll create an MVC Controller. Twilio will call our webhook once an SMS text message has been received.
Right click the Controllers folder in your project, select Add > Controller and add an empty MVC 5 Controller. You can name your Controller as you see fit, in this example we called it MessagingController.
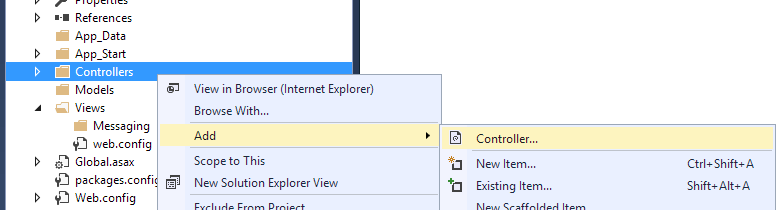
Next you will need to add the using statements to import the Twilio namespaces;
using Twilio.AspNet.Common; using Twilio.AspNet.Mvc; using Twilio.TwiML;
and change the Controller class to inherit from TwilioController.
Now we add the following code;
[HttpPost] [IgnoreAntiforgeryToken] public TwiMLResult Index(SmsRequest request) { var response = new MessagingResponse(); response.Message("Hello World"); return TwiML(response); }
Notice the use of the [IgnoreAntiforgeryToken] attribute, this is to allow 3rd parties (like Twilio or whoever) to send a POST to the API method.
Of course you can code your webhook however you need but for our example, we have our response message set as “Hello World” so when our webhook receives notification of a SMS text to our Twilio number, it will respond with our own message.
Run the Application
So now we can go ahead and run our application. As this is a simple project with the only content being our Controller, you will see an error page. That doesn’t matter, we just need our project to be running in Visual Studio so we can make use of the webhook.
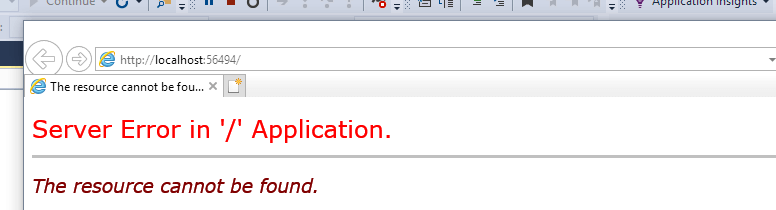
Use Conveyor
Now the application is running, we can use Conveyor to obtain a publicly accessible URL that we can provide to Twilio. Clicking Access Over Internet in the Conveyor window will then provide us with the URL we need. You may need to register an account at this point.
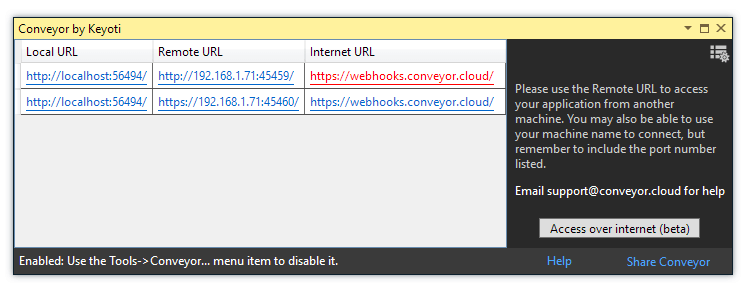
Calls to the conveyor.cloud URL are tunnelled to your localhost, so we will add the Controller name to the conveyor.cloud URL and then add it to the Twilio settings.
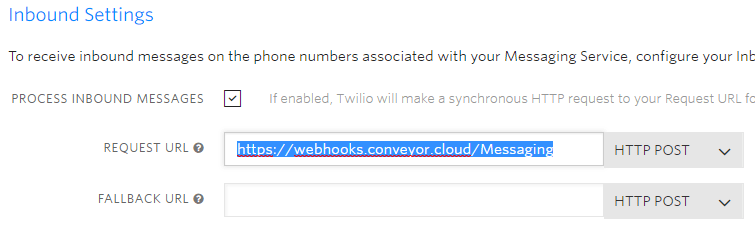
That’s it. We’re ready to test it out!
Now when I send a SMS text to my Twilio number the message from our application is sent back to the original mobile number.
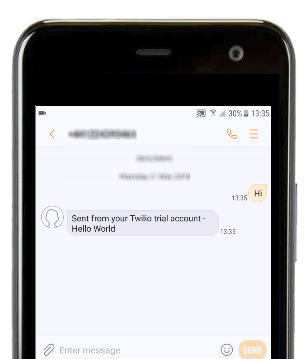
Conclusion
As Conveyor tunnels through your firewall and handles all the configuration settings, it makes it extremely easy to setup direct access to your web application.
In the same way, Conveyor can be used to test your application from various devices, either from your Local Area Network or over the internet.