Working with Slack Webhooks from Localhost - ASP.NET MVC Tutorial
Slack Webhooks, directly on localhost
We are going to show you how to setup a simple webhook scenario with Slack but also how to test and work with your Slack webhook directly from your Visual Studio development server.
For our example, we’ll be setting up Slack to post to our web app once a trigger word is entered at the begining of a message. Our web project will receive the post and return a message directly to our Slack conversion. All without having to deploy to a web host or Azure.
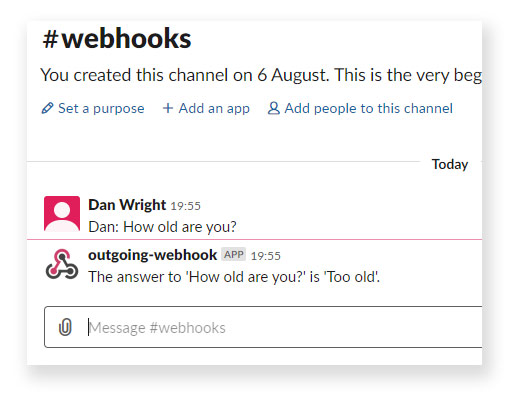
Create your web project
So to get started let's create an empty C# web application with Web API in Visual Studio which we will use for receiving WebHooks from Slack.
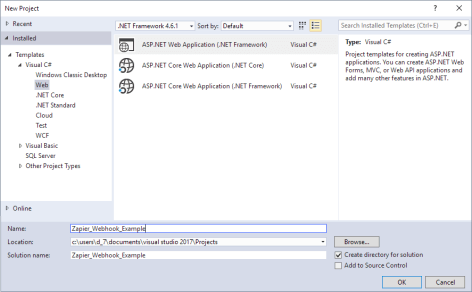
We don’t need to select Azure since we’ll be showing you how to open up IIS Express directly over the internet.
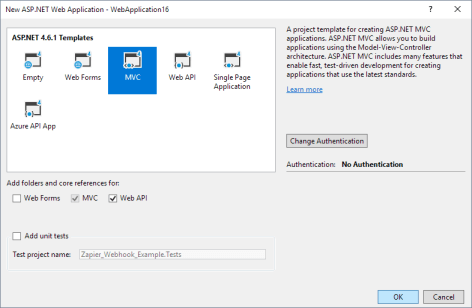
You will need to install the following Nuget package;
Microsoft.AspNet.WebHooks.Receivers.Slack
Then initialize the Slack webhook receiver in the WebApiConfig method as below:
public static class WebApiConfig { public static void Register(HttpConfiguration config) { // Web API configuration and services // Web API routes config.MapHttpAttributeRoutes(); config.Routes.MapHttpRoute( name: "DefaultApi", routeTemplate: "api/{controller}/{id}", defaults: new { id = RouteParameter.Optional } ); // Load Slack receiver config.InitializeReceiveSlackWebHooks(); } }
Set a secret key
To authenticate the incoming Slack webhook we set a token under the root Web.config application settings like this;
<appSettings> <add key="MS_WebHookReceiverSecret_Slack" value="yourToken" /> </appSettings>
We will grab the token id from the Slack webhook setup and add it above in a moment.
Configuring Slack WebHook Handler
Now we need to add the file, SlackWebHookHandler.cs to the project. This will hold the following class which will handle the incoming Slack webhook
public class SlackWebHookHandler : WebHookHandler { public override Task ExecuteAsync(string generator, WebHookHandlerContext context) { NameValueCollection nvc; if (context.TryGetData<NameValueCollection>(out nvc)) { string question = nvc["subtext"]; string msg = string.Format("The answer to '{0}' is '{1}'.", question, "Too old"); SlackResponse reply = new SlackResponse(msg); context.Response = context.Request.CreateResponse(reply); } return Task.FromResult(true); } }
Setting Up Slack Webhook
Head on over to Slack and click on Apps. You're looking for Outgoing Webhooks. Install the app.
Now we just need to configure it. Choose a specfic channel to listen to, or select All to listen to every Slack channel.
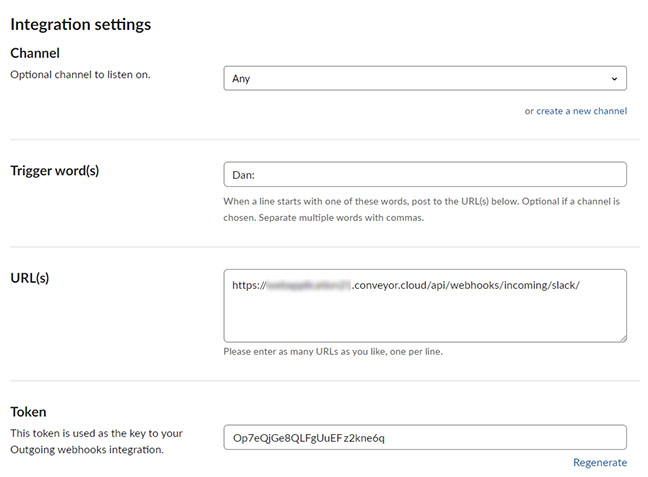
Next, enter the trigger words to initiate the webhook. This is the first word of a conversion - it can be anything you like.
Now we need to enter the URL to your application. We will be using the Conveyor Visual Studio Extension which will tunnel through the firewall and to the web development server, providing external access to our web application. If you haven't already installed Conveyor, you can find it for free in the Visual Studio Extensions Marketplace, directly in Visual Studio, or here. Once installed, make sure to run your application and within the Conveyor window enable Access Over Internet. You will need to register for an account if you haven't already. Conveyor will provide you with an internet URL for you to use to reach your web app.
When you run your project, the Conveyor window will show you the URL for accessing your application. Just click on Access Over Internet and login. Conveyor will then display the Internet URL.
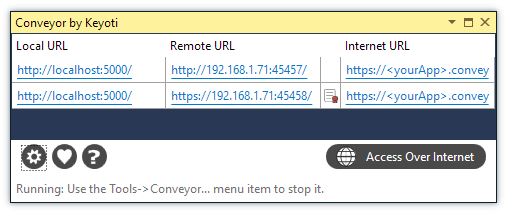
In the Slack webhooks settings, under URLs, enter <ConveyorURL>/api/webhooks/incoming/slack/
Grab the Token and add it to your Web.config as shown earlier in this tutorial. You can now Save your settings and return to Slack.
All Set!
That's it. Just make sure your application is running in Visual Studio, along with Conveyor.
Send a message on Slack with your trigger word as the first word. You should receive a response via your application - pretty cool!
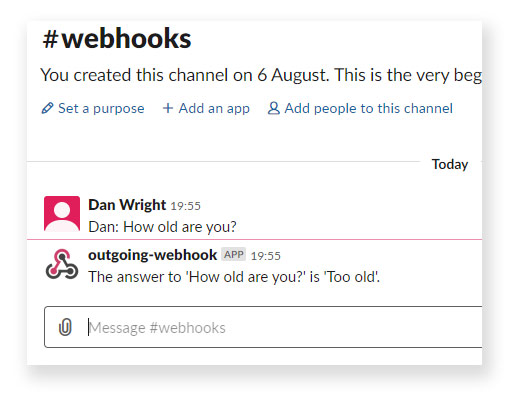